Monitor Server with Telegram Bot and Python
Build a bot to monitor the server using Python and Telegram.
The server usually must be active 24/7 and in good condition. So, ideally, the server must be monitored to avoid incidents.
What if we have monitoring tools in our smartphones? For instance, while chatting with someone whenever we want to check the server, we could just type some command in our smartphone and all information would be sent to us.
Today, we wanna make such a tool using Python and Telegram. And at no cost.
Okay, let’s build the tool…
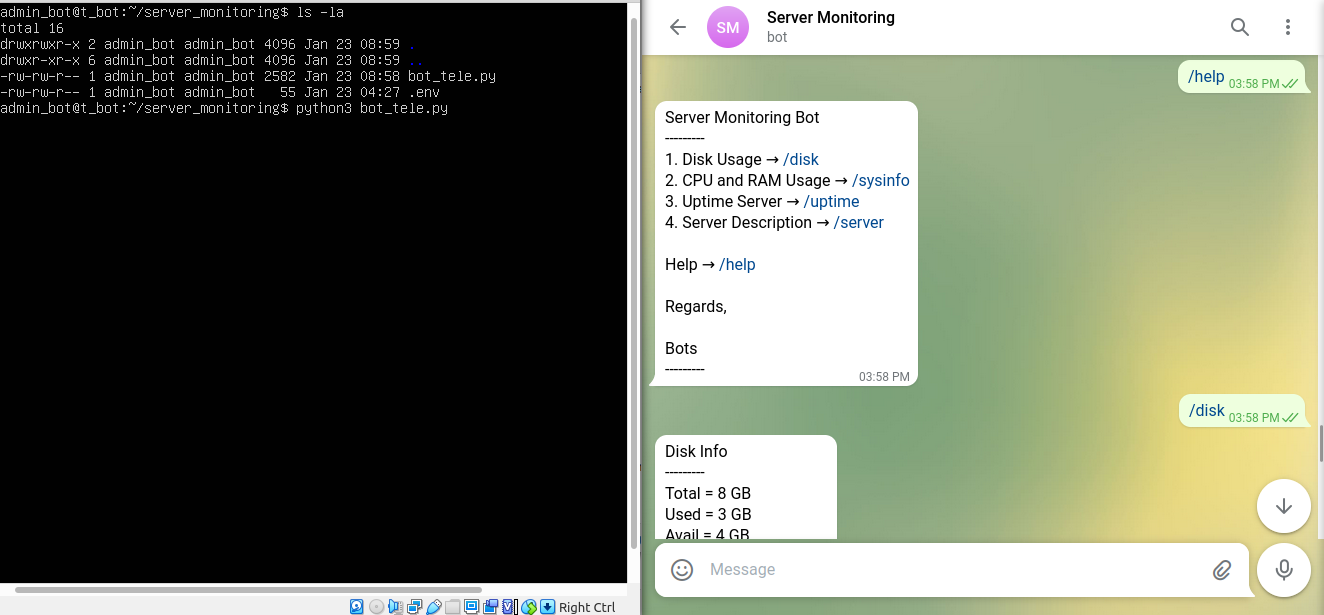
Step 1. Create Telegram Bot
Create a new account in telegram, and chat with BotFather to register a newbot and get the token to access.
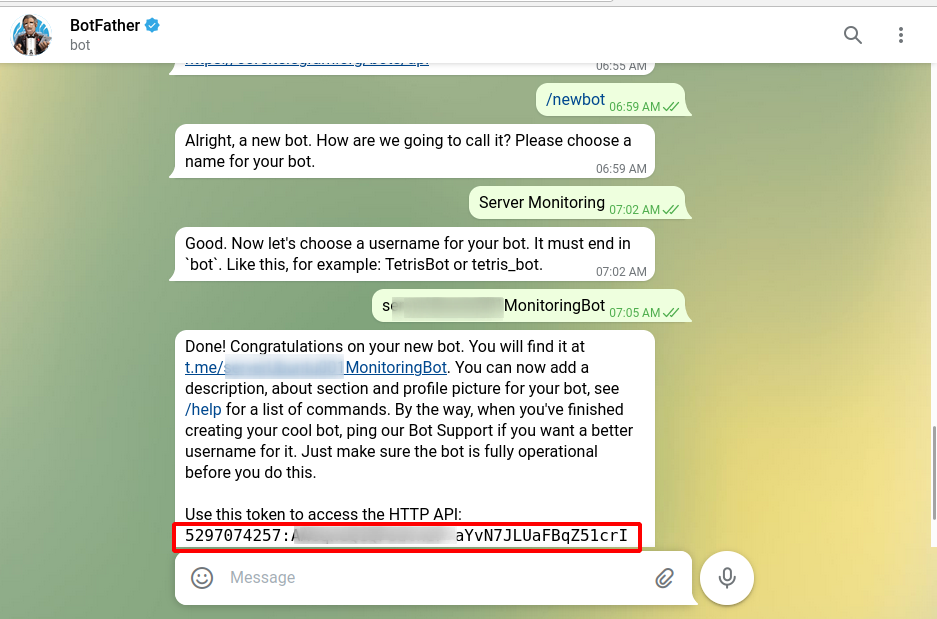
Step 2 — Prepare What You Want to Monitor
In this simple project, I wanna monitor 4 types of data, and if you want more, just Google it and add it to your code;
1. Disk Usage
2. CPU and RAM Usage
3. Uptime Server
4. Server Description (OS info, hostname, IP address)
How to Collect Data from Server
I use a powerful package in Python to get any information about the system, psutil. Also, I use subprocess to run some Linux commands.
So the first task is to import the package:
import psutil
import subprocess
- Disk Usage
diskTotal = int(psutil.disk_usage('/').total/(1024*1024*1024))
diskUsed = int(psutil.disk_usage('/').used/(1024*1024*1024))
diskAvail = int(psutil.disk_usage('/').free/(1024*1024*1024))
diskPercent = psutil.disk_usage('/').percent
- CPU and RAM Usage
cpuUsage = psutil.cpu_percent(interval=1)
ramTotal = psutil.virtual_memory().total/(1024*1024*1024) #GB
ramUsage = psutil.virtual_memory().used/(1024*1024*1024) #GB
ramFree = psutil.virtual_memory().free/(1024*1024*1024) #GB
ramUsagePercent = psutil.virtual_memory().percent
- Uptime Server
upTime = subprocess.check_output(['uptime','-p']).decode('UTF-8')
- Server Description
uname = subprocess.check_output(['uname','-rsoi']).decode('UTF-8')
host = subprocess.check_output(['hostname']).decode('UTF-8')
ipAddr = subprocess.check_output(['hostname','-I']).decode('UTF-8')
Step 3. Make a Connection with Telegram Bot
From here, we will be making a simple connection to Telegram Bot and managing API KEY in python code securely.
- Install pyTelegramBotAPI and python-dotenv
sudo apt-get install python3-pip
pip3 install pyTelegramBotAPI
pip3 install python-dotenv
- Create file .env to save API KEY (token)
API_KEY=<your token>
- Simply get a message and reply to Telegram chat
import os
import telebot
from dotenv import load_dotenv# load API_KEY from .env
load_dotenv()
API_KEY = os.getenv("API_KEY")# make a connection to bot
bot = telebot.TeleBot(API_KEY)# make command function and bot will reply the command
@bot.message_handler(commands=['hello'])
def hello(message):
bot.reply_to(message, "Hi, I'am Server Monitoring Bot")# make command function to chat if command receive
@bot.message_handler(commands=['check'])
def check(message):
bot.send_message(message.chat.id, "The server is alive")# listen to telegram commands
bot.polling()
- Testing
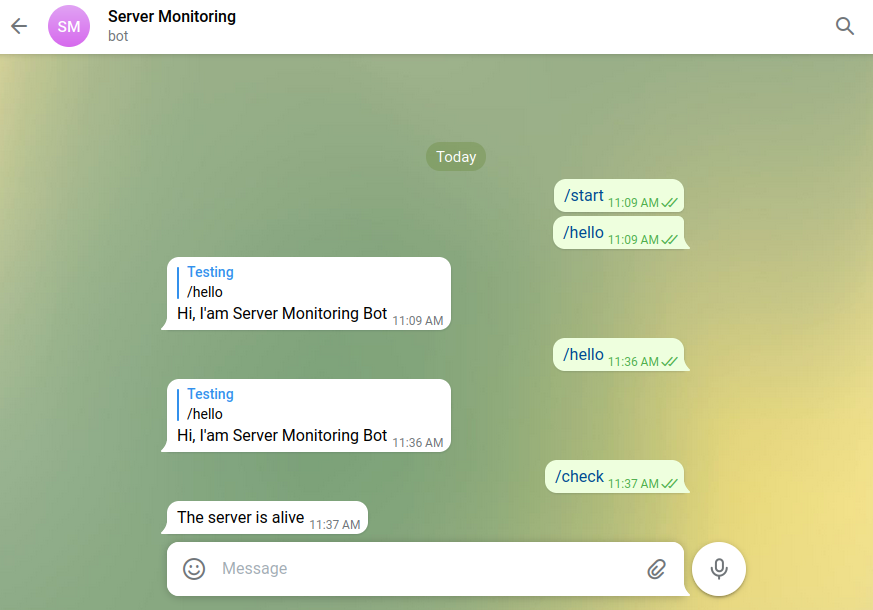
Step 4. Combine Telegram Bot and Monitoring Server
First of all, we must define the command to connect from Telegram to the server. /start or /help will send this message:
Server Monitoring Bot
---------
1. Disk Usage → /disk
2. CPU and RAM Usage → /sysinfo
3. Uptime Server → /uptime
4. Server Description → /serverHelp → /helpRegards,Bots
---------
Development
The idea is just to make the program understand commands and send data back to telegram chat. We have a connection with Telegram Bot and we have data from the server. Just make it happen. And if you are so lazy, copy and paste my code to your machine. But, make sure that you understand what you write.
Step 5. Run the Program (Final)
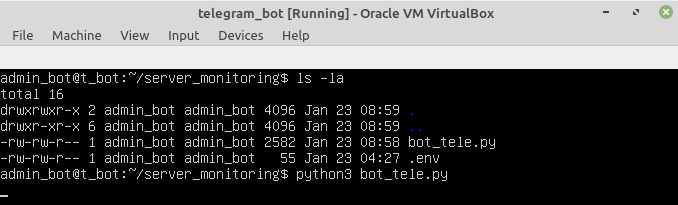
Interact with Server Monitoring (bot) in Telegram apps.
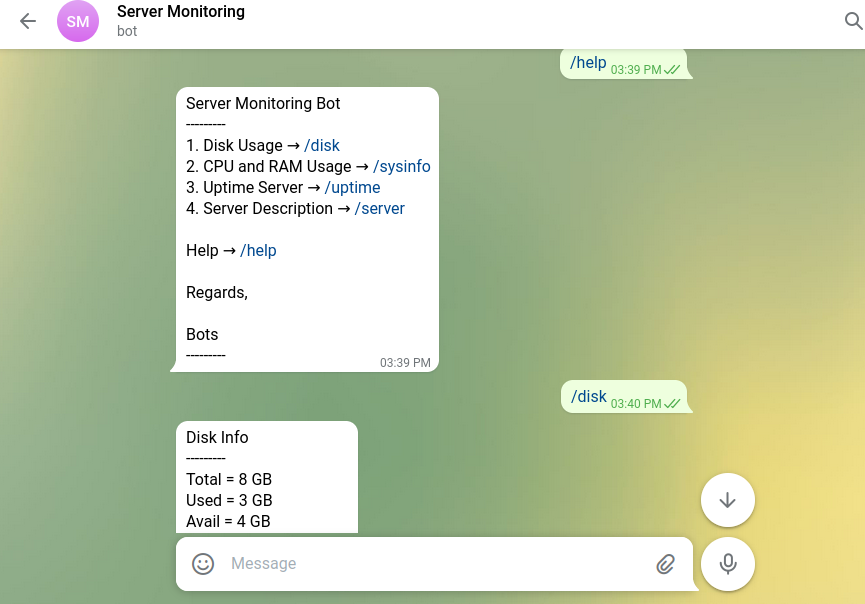
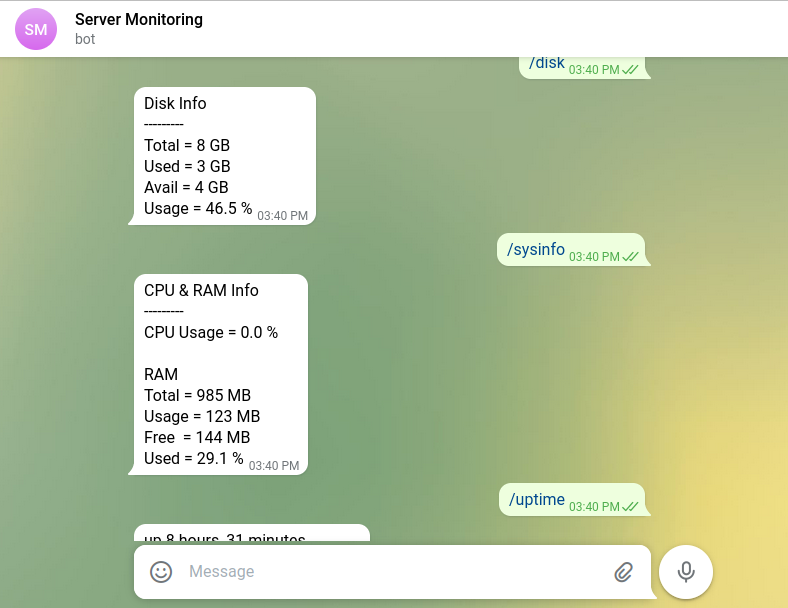
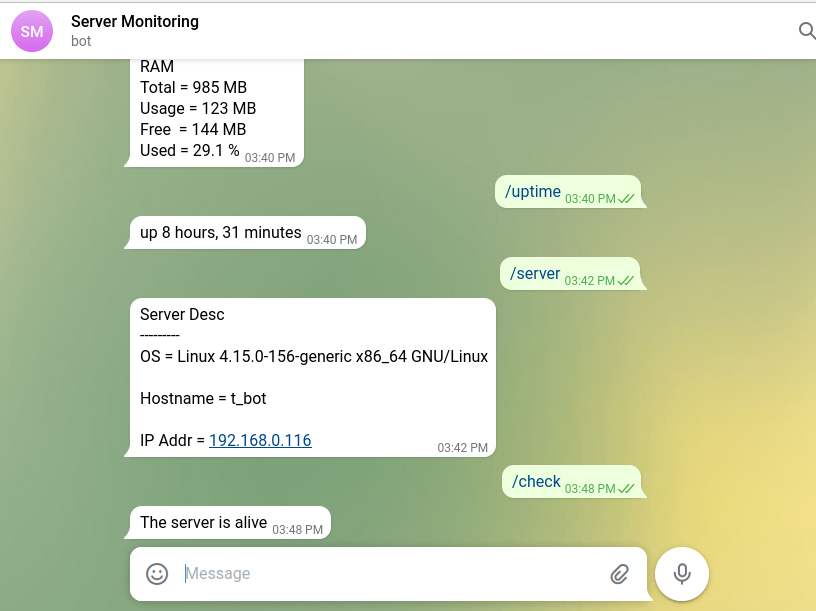
(Bonus) Troubleshoot and Make Autorun
- Ubuntu 18.04 can’t get repository
I use Ubuntu 18.04 and have problems when using apt-get update or apt install pip. This problem comes when the server repo is not reachable.
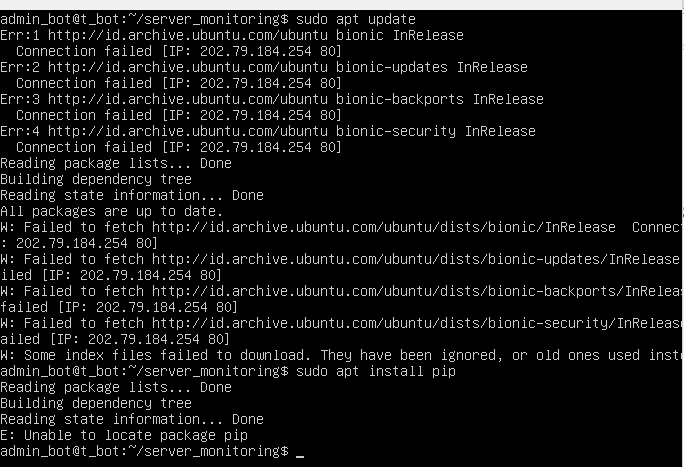
So I change to the local mirror (Indonesia’s repository), and put this text to /etc/apt/sources.list:
deb http://buaya.klas.or.id/ubuntu bionic main restricted universe multiverse
deb http://buaya.klas.or.id/ubuntu bionic-updates main restricted universe multiverse
deb http://buaya.klas.or.id/ubuntu bionic-security main restricted universe multiverse
deb http://buaya.klas.or.id/ubuntu bionic-backports main restricted universe multiverse
deb http://buaya.klas.or.id/ubuntu bionic-proposed main restricted universe multiverse
And it works!
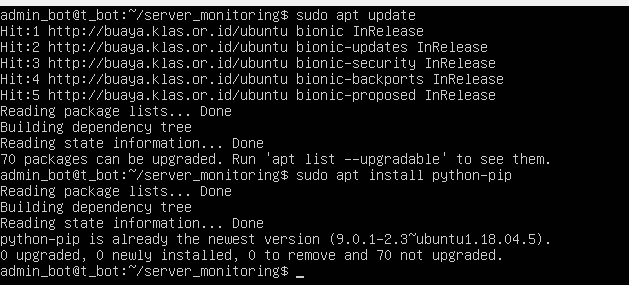
- Make the program auto-run when the server is active
open the crontab -e, add this code to the end of the file:
@reboot python3 /home/admin_bot/server_monitoring/bot_tele.py
Conclusion
Today we successfully made a Telegram Bot with python for monitoring the server, congratulation. Don’t stop from here, explore your imagination, and amaze the world.
Thanks for reading.
More content at plainenglish.io. Sign up for our free weekly newsletter. Get exclusive access to writing opportunities and advice in our community Discord.
Ten articles before and after
How to create a Telegram bot with Python in under 10 min! – Telegram Group
Телеграм бот для уведомлений об ордерах Binance – Telegram Group
IntruderDet —An Intruder Detection Bot for my Appartment – Telegram Group
Send APK automatically to Telegram using Dart/Flutter – Telegram Group
How to build a Telegram bot to show Chainlink price feeds – Telegram Group
Google Sheets Formula & Telegram Message ✈️ – Telegram Group
How to Create a Telegram Chatbot in 2022 – Telegram Group
Telegram Bot 跟我想的不一樣. 第一天進公司時,Slack就被邀進同事們訂便當的群組,在上面會宣布今天訂那一家便… – Telegram Group
TRX1 Dev Blog #11 (November 2021) – Telegram Group
Serverless Telegram bot with Kotlin, Firebase and Google Cloud Functions – Telegram Group