How to create a telegram bot in GOLANG
Telegram is a messenger app that is growing more every day. It has tons of features including bots. Bots can interact with users, groups, supergroups and channels.
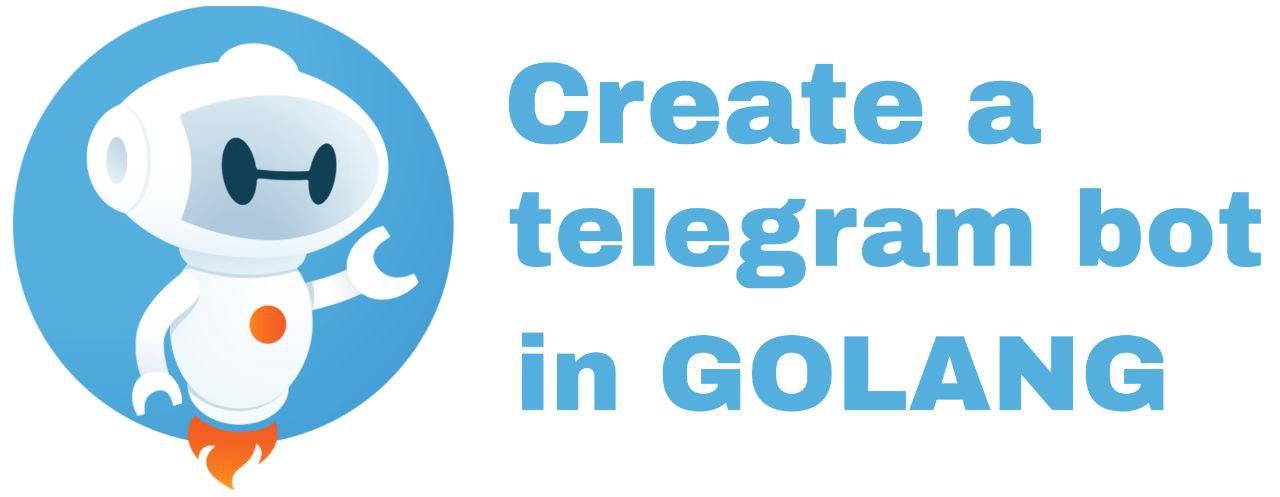
In this article, we are going to learn how to create a telegram bot in golang.
Requirements
- Telegram account : You need a telegram account for creating the bot using botfather (described later).
- telego module : Telego is a new library for creating telegram apps in golang.
Installing the module
To install telegot you need to install it into your $GOPATH using go get command. Just type the following in your terminal to install it :
go get -u github.com/SakoDroid/telego
Creating the bot
First you need to create a new bot using bofather. Steps below will show you how to do it :
- Start the botfather bot.
- Send /newbot command to botfather.
- Choose a unique name for your bot.
- If everything is ok you will receive a message like a picture below from botfather. It contains a token which we will use later so write the token somewhere.

Now you have created the bot. Lets move to the coding part.
Writing the code
To write the code for the bot in golang we will use telego library as mentioned earlier.
We will go through this section step by step :
step 1: importing libraries. Just import the following libraries :
package main
import (
bt "github.com/SakoDroid/telego"
cfg "github.com/SakoDroid/telego/configs"
objs "github.com/SakoDroid/telego/objects"
)
step 2: Creating the bot configs. Bot configs is a struct that has some fields for configuring bot’s actions. These configs are located in configs package which is imported as cfg. This package has some default values if you don’t wanna mess with the configs. Bot configs have a field called UpdateConfigs which has the configurations related to requesting and receiving updates from the API server. Now let’s take a look at the code :
package mainimport (
bt "github.com/SakoDroid/telego"
cfg "github.com/SakoDroid/telego/configs"
objs "github.com/SakoDroid/telego/objects"
)//This the token you receive from botfather
const token string = "5078468473:AAHLCQfMnJTIFM25rFlbU2k5432kYD46dhs"//The instance of the bot
var bot *bt.Botfunc main() { //Update configs
up := cfg.DefaultUpdateConfigs()
//Bot configs
cf := cfg.BotConfigs{
BotAPI: cfg.DefaultBotAPI,
APIKey: token, UpdateConfigs: up,
Webhook: false,
LogFileAddress: cfg.DefaultLogFile
}
}
About the BoConfigs fields :
1. APIKey: This is the token you received from botfather when you created the bot.
2. BotAPI: This is the address of the bot API server. If you are not running a local api server, use cfg.DefaultBotApi constant for this field.
3. UpdateConfigs: This is the update related configs such as update frequency (default : 300 milliseconds). You can use cfg.DefaultUpdateConfigs() method for this field.
3. Webhook: Pass true if you want to use webhook for receiving updates. (Telego currently does not support webhook).
4. LogFileAddress: The logs of the bot will be saved in this file.
Step 3: Creating the bot instance. Now that we have created the configs it’s time to create the bot. Just pass the configs you have created to NewBot method of the telego package ( imported as bt ). After you have created the bot. you need to call the Run() so that the bot starts and begins receiving updates from the API server.
package mainimport (
"fmt"
bt "github.com/SakoDroid/telego"
cfg "github.com/SakoDroid/telego/configs"
objs "github.com/SakoDroid/telego/objects"
)const token string = "5078468473:AAHLCQfMnJTIFM25rFlbU2k5432kYD46dhs"//The instance of the bot.
var bot *bt.Botfunc main() { up := cfg.DefaultUpdateConfigs()
cf := cfg.BotConfigs{
BotAPI: cfg.DefaultBotAPI,
APIKey: token, UpdateConfigs: up,
Webhook: false,
LogFileAddress: cfg.DefaultLogFile
}
var err error
//Creating the bot using the created configs
bot, err = bt.NewBot(&cf)
if err == nil {
//Start the bot.
err = bot.Run()
if err == nil{
start()
}else {
fmt.Println(err)
}
} else {
fmt.Println(err)
}
}func start(){
//Described in later sections
}
Step 4: Receiving updates and interacting with clients. Telego offers two ways for handling received updates from API server :
- Handlers
- Go channels
- Handlers: Handlers can be used for text messages and callback queries (A type of inline keyboard buttons). You specify a regex pattern, chat types and a function. Every time a text message is received from the specified chat types the function will be called with the update passed into it. Chat types can be “private”, “group”, “supergroup”,channel” and “all”. You can specify multiple chat types. Let’s add a handler for text message “/start” and respond “Hi i’m a telegram bot!” :
package mainimport (
"fmt"
bt "github.com/SakoDroid/telego"
cfg "github.com/SakoDroid/telego/configs"
objs "github.com/SakoDroid/telego/objects"
)const token string = "5078468473:AAHLCQfMnJTIFM25rFlbU2k5432kYD46dhs"//The instance of the bot.
var bot *bt.Botfunc main() { up := cfg.DefaultUpdateConfigs()
cf := cfg.BotConfigs{
BotAPI: cfg.DefaultBotAPI,
APIKey: token, UpdateConfigs: up,
Webhook: false,
LogFileAddress: cfg.DefaultLogFile
}
var err error
//Creating the bot using the created configs
bot, err = bt.NewBot(&cf)
if err == nil {
err = bot.Run()
if err == nil{
start()
}else {
fmt.Println(err)
}
} else {
fmt.Println(err)
}
}func start(){
bot.AddHandler("/start", func(u *objs.Update) {
//Sends the message to the chat that the message has been received from. The message will be a reply to the received message.
_,err := bot.SendMessage(u.Message.Chat.Id,"hi i'm a telegram bot!","",u.Message.MessageId,false,false)
if err != nil{
fmt.Println(err)
}
}, "private","group")
}
Since we have passed “private” and “group” to the AddHandler method, this handler will only work if the received message is from a private chat or a group chat.
2. Go channels: In Telego you can register channels for specified chats and update types. Update type is the file that an update contains. It can contain message, edited_message, inline_query, etc . You can register a channel which will be updated when an update contains the specified field. You can specify a chat too, meaning the channel will be updated when it’s from that chat. This two parameters can be combined together to create channels that will be dedicated to a certain update type in a specified chat.
In the code below we register a channel for only receiving message updates. Since we don’t specify a chat id, all the updates from all chats that contain message field will be passed into this channel . Let’s take a look :
package mainimport (
"fmt"
bt "github.com/SakoDroid/telego"
cfg "github.com/SakoDroid/telego/configs"
objs "github.com/SakoDroid/telego/objects"
)const token string = "5078468473:AAHLCQfMnJTIFM25rFlbU2k5432kYD46dhs"//The instance of the bot.
var bot *bt.Botfunc main() { up := cfg.DefaultUpdateConfigs()
cf := cfg.BotConfigs{
BotAPI: cfg.DefaultBotAPI,
APIKey: token, UpdateConfigs: up,
Webhook: false,
LogFileAddress: cfg.DefaultLogFile
}
var err error
//Creating the bot using the created configs
bot, err = bt.NewBot(&cf)
if err == nil {
err = bot.Run()
if err == nil{
start()
}else {
fmt.Println(err)
}
} else {
fmt.Println(err)
}
}func start(){ //Register the channel
messageChannel, _ := bot.AdvancedMode().RegisterChannel("", "message")
for {
//Wait for updates
up := <- *messageChannel
//Print the text
fmt.Println(up.Message.Text)
}
}
This code will receive updates through messageChannel and print the text.
Interacting with the user
Now that we know how to receive updates, how can we send messages, media and other stuff back to the user?
Telego has a lot of methods for sending back text, photo, video, sticker, etc. They all start with Send , like SendMessage which will send a text message or SendPhoto which will send a photo.
For sending medias, calling the related method will return a MediaSender. Based on telegram bot API documentation, medias can be sent in three ways :
- Using file id of a file which is already stored in telegram servers.
- Using an HTTP url.
- Using a file that is in your computer.
MediaSender covers all these three ways. It has two methods : SendByFileIdOrUrl and SendByFile .
SendByFileIdOrUrl will take a string which is either a file id or a URL. SendByFile takes a file and uploads it to telegram servers.
** Since all the send methods return the sent message, you can save the file id that is in the returned message for further use and avoid uploading the media every time.
(For sending other message like polls and locations you can read the full documentation of Telego here )
Now let’s create a code that will send two pictures every time text message “pic” is received. One of them is an HTTP URL and the other on is file stored in the computer that the bot is running on. We will use handlers in this case ( other parts of the code are shown above and are deleted here ) :
func start(){
bot.AddHandler("pic", func(u *objs.Update) {
//ChatId of this chat
chatId := u.Message.Chat.Id //Received message if. We will use it's id to reply to it
messageId := u.Message.MessageId //Create a media sender for sending a URL
mediaSender1 := bot.SendPhoto(chatId,messageId,"this is the caption","")//Send the URL
_,err := mediaSender1.SendByFileIdOrUrl("http://example.com/funny_cat.jpg",false,false)
if err2 != nil{
fmt.Println(err)
} //Create a media sender for sending a file
mediaSender2 := bot.SendPhoto(chatId,messageId,"this is the caption","") //Open the file. error is ignored to simplify the code
file,_ := os.Open("funny_cat.jpg")//Send the file
_,err = mediaSender2.SendByFile(file,false,false) if err != nil{
fmt.Println(err)
}
}, "private")
}
And that’s it, you just sent two photos!
The code for sending other medias is like the code above but you just have to call the related method of the bot ( like SendVideo or SendGif ).
About keyboards
Telegram offers two types of keyboards :
- Custom keyboards : This keyboards will be shown to the user(s) instead of the letter keyboard. (You can switch between them)
- Inline keyboards : This keyboard will be shown below the message it has been sent with.
Telego has a too for creating both keyboards and it’s really easy to use. For creating a custom keyboard you need to call CreateKeyboard method and for creating an inline keyboard you can call CreateInlineKeyboard method. This methods will return a keyboard pointer which has several methods for adding buttons to them. After you have completed the keyboard you can send them to the user(s) along with a message. For sending them, just pass the keyboard for keyboard argument of the Send methods of the advanced bot.
We will cover them both with example codes.
Custom keyboards
The code below creates a custom keyboard and sends it with a text message :
func start(){
bot.AddHandler("/start", func(u *objs.Update) {
//Create the custom keyboard
kb := bot.CreateKeyboard(false, false, false, "type ...") //Add buttons to it. First argument is the button's text and the second one is the row number that the button will be added to it. kb.AddButton("button1", 1) kb.AddButton("button2", 1)kb.AddButton("button3", 2)
//Pass the keyboard to the send method
_, err := bot.AdvancedMode().ASendMessage(u.Message.Chat.Id, "hi to you too, send me a location", "", u.Message.MessageId, false,false, nil, false, false, kb) if err != nil { fmt.Println(err) } }, "private","group")
}
When each of this buttons are pressed, a text message containing the text of the button is sent to the bot. So for handling each button you can add a handler for the text the button holds.
Inline keyboards
Inline keyboards have several types of buttons. Telego has methods for adding all of these buttons. The methods are fully documented in the source code. Now let’s create an inline keyboard :
func start(){ bot.AddHandler("/start", func(u *objs.Update) { //Creates a inline keyboard
kb := bot.CreateInlineKeyboard() //Adds a url button, when this button is pressed the url will be opened.
kb.AddURLButton("url", "https://google.com", 1) //Adds a button which will send back a callback query containing the data we give to this function, to the bot when it's pressed.
kb.AddCallbackButton("call back without handler", "callback data 1", 2) //Adds a button which will send back a callback query containing the data we give to this function, to the bot when it's pressed. The difference between this method and the last method is that this method adds a handler for the callback of this button.
kb.AddCallbackButtonHandler("callabck with handler", "callback data 2", 3, func(u *objs.Update) { _, err3 := bot.AdvancedMode().AAnswerCallbackQuery(u.CallbackQuery.Id, "callback received", true, "", 0) if err3 != nil { fmt.Println(err3) } }) //Pass the keyboard to the send method
_, err := bot.AdvancedMode().ASendMessage(u.Message.Chat.Id, "hi to you too, send me a location", "", u.Message.MessageId, false,false, nil, false, false, kb) if err != nil { fmt.Println(err) } }, "private","group")
}
In this article, we covered the basics of creating a bot using telego library in golang. For further reading, you can read the telego documentation to know more about creating a bot using telego library in golnag.
Ten articles before and after
Telegram iOS Translate Feature — How the hell did they do it? – Telegram Group
YGG SEA Sticker Contest. We have an exciting competition for… – Telegram Group
Digital Privacy: A Myth or Reality..? – Telegram Group
Как закрепить сообщение в Телеграм. Что нужно сделать? – Telegram Group
How to install Telegram on Ubuntu 20.04 – Telegram Group
Smart Door Lock System using esp32-cam and Telegram chatbot – Telegram Group
Minipod do Telegram – Telegram Group
An overview of Telegram monetization efforts – Telegram Group
Translate text through Google Translate using Python – Telegram Group