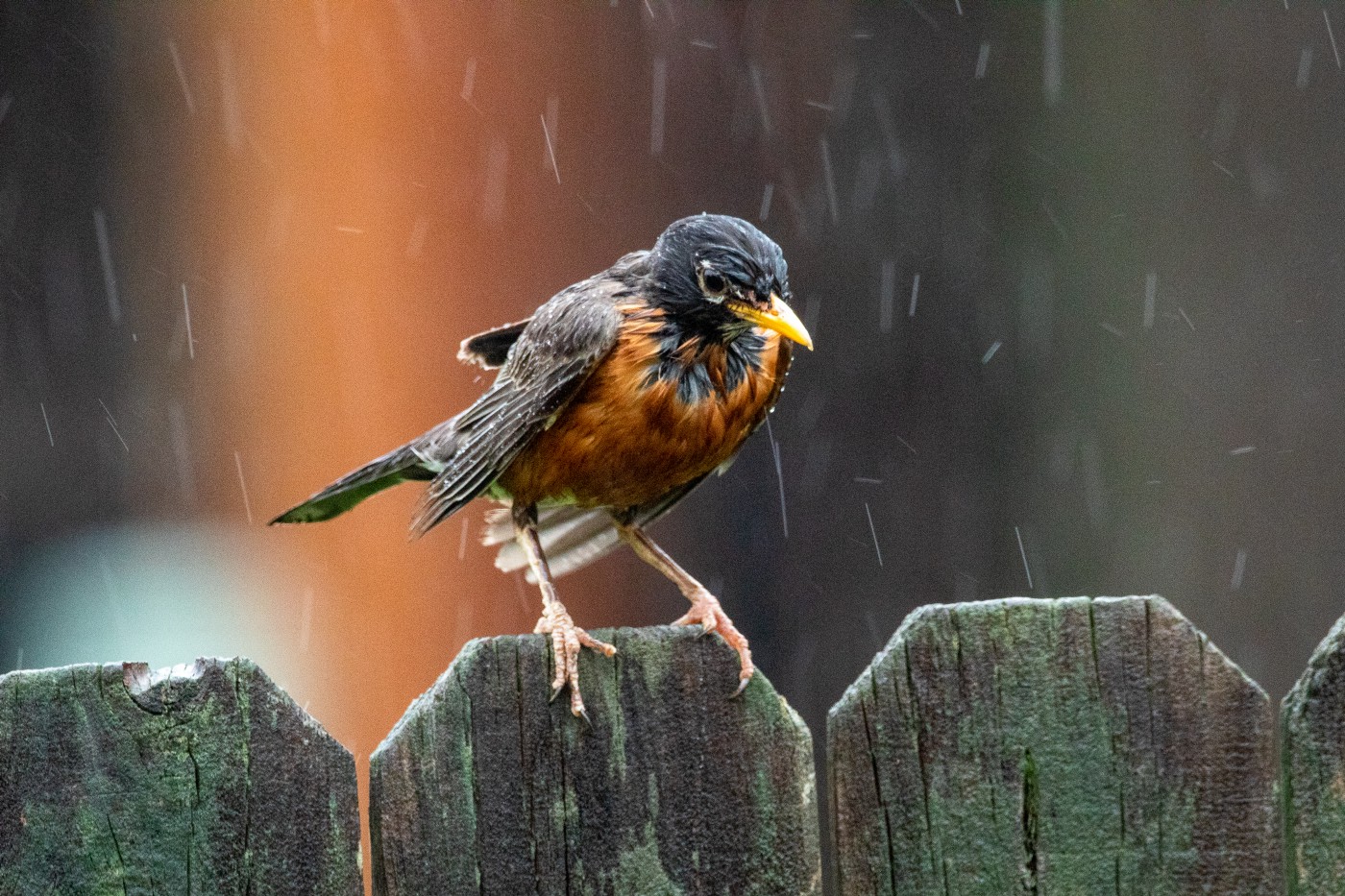
Stream Tweets to a Telegram channel
Streaming Tweets to a TG channel is a common task. Here comes a short tutorial on how to built a minimal bot that listens for Tweets containing predefined strings and forwards them to a channel for your choice.
Let’s start.
At first we want to get our stream to work. To this end, we use the tweepy library. If you haven’t installed tweepy yet, you can easily get it via pip. Just run
pip install tweepy
in your console. This installs the latest release from PyPi. At the time I’m writing this article, this would install version 3.10.0. This tutorial assumes that you have that version (or lower) installed. If you have already worked with tweepy streams however, you may have stumbled across an error like this: ProtocolError: (‘Connection broken: IncompleteRead(0 bytes read)’, IncompleteRead(0 bytes read)). The latest development version of tweepy (at this point in time) handles that error and can be downloaded from git directly via
pip install git+https://github.com/tweepy/tweepy.git
Now that tweepy is installed, we can make use of the “StreamListener”-class.
To implement our own Listener, we write a class that inherits from “StreamListener” and overwrite some of its methods to cater for our needs. Of course we need to import tweepy along with some other usefull stuff like logging and json first.
import os
import json
import loggingimport tweepyclass MyListener(tweepy.StreamListener):
def __init__(self):
self.logger = logging.getLogger("MyListenerLogs") self.logger.setLevel(logging.DEBUG) def on_connect():
self.logger.info("connected to stream !")
So far so good. We defined the Listener class and set up a logger with a name parameter so that we can tell apart the logs from our listener and the TG bot logs later on.
Right after that, we implemented the “on_connect”-method which gets called once a connection to the stream has been established. In our case, we just log a message; but you could do pretty much anything here (which also holds true for all other methods of the listener class).
Next we want to get some real Twitter data. We continue the above snippet by implementing the “on_data”-method. This method gets invoked, when ever we receive raw data from the stream. This is the place where all the bot-logic sits.
def on_data(self, status):
tweet = json.loads(status) tweet_text = tweet["text"]
tweet_id = tweet["id"]
tweet_url = f"https://twitter.com/twitter/statuses/{tweet_id}"
Now comes the bot-logic. The lib. we use to interface with the TG bot API is “python-telegram-bot”.
pip install python-telegram-bot
Note: The name of the lib is “python-telegram-bot” but for the import, we have to use “telegram”.
import os
import json
import loggingimport tweepy
import telegram # <---
Having telegram available, we can continue to work on the “on_data”-method we started.
def on_data(self, status):
tweet = json.loads(status) tweet_text = tweet["text"]
tweet_id = tweet["id"]
tweet_url = f"https://twitter.com/twitter/statuses/{tweet_id}" try:
bot = Bot(token = <the token you obtained from botfather>)
bot.send_message(
chat_id = "@name_of_your_channel",
text = tweet_text
)
except:
logger.error("faild forwarding tweet to channel")
Ok “on_data” is ready. Now we need a place where we can run the stream. Right below the listener-class we just wrote, we define a function “main”, where we instanciate the listener and do all the other telegram-related stuff.
def main():
token = os.environ.get("token") # for production
updater = telegram.Updater(token = token)
listener = MyListener
strean = tweepy.Stream(
auth = api.auth, # we'll come to that
listener = listener()
)
stream.filter(track = ["@FOO", "BAR", ...])
updater.start_polling()
updater.idle()if __name__ == "__main__":
main()
Alright. We have our listener, the bot-logic and all of it wrappend by the “main”-function. The only thing that’s still missing it authentication with Twitter. This tutorial assumes that the reader has his credentials obtained already. The authentication process is fairly straight-forward. Just do:
consumer_key = ""
consumer_secret = ""
access_token = ""
access_token_secret = ""auth = tweepy.OAuthHandler(
consumer_key,
consumer_secret
)auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
. Good ! That’s it. I hope this tutorial was helpful and a clap would be very much appreciated ! Thanks and bye !
Ten articles before and after
Django Telegram Bot. В данной статье я расскажу о том как… – Telegram Group
data-rh=”true”>Telegram Bot for Lazy – Max Makhrov – Medium – Telegram Group
Домашняя бухгалтерия в telegram. Начало. – Telegram Group
Telegram bot in Go app. Notifications are important part of any… – Telegram Group
Monitorar suas aplicações na AWS usando o Telegram – Telegram Group
Written in collaboration with Sushil Shaw – Telegram Group
How I Connected with 10,000 people over a couple of weeks – Telegram Group
python Telegram bot pytelegrambotapi telegram bot simple – Telegram Group
Bot sederhana dengan menggunakan python! – Telegram Group
Telegram Secret Chat — Enable Now to be Secure – Telegram Group