Creating a Bot using the Telegram Bot API
(In this part, we shall create our first simple Telegram Bot and use the Go programming language to access it too)
Preamble
Telegram is a messaging app which is super-fast, simple, secure and free.
I first installed the Telegram app on my Android phone. I also installed their native app on my Windows 7 Professional desktop.
Telegram has an open API and protocol free for everyone. With this API we can build our own tools.
Objective
To create a simple bot (called SMTFirstBot) using their open API.
What’s a Bot?
Bots are third-party applications that run inside Telegram. Users can interact with bots by sending them messages, commands and inline requests. You control your bots using HTTPS requests to their bot API. Telegram bots are special accounts that do not require an additional phone number to set up. Users can interact with bots.
How do I create a bot?
There’s a… bot for that.
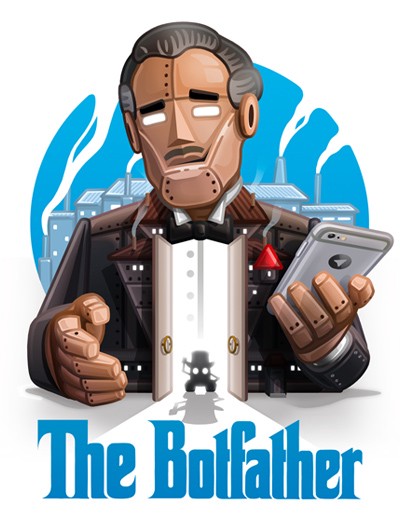
Just talk to @BotFather and follow a few simple steps.
It opened a separate window on my Telegram native app.
Next I typed:
You: /newbot
BotFather: Alright, a new bot. How are we going to call it? Please choose a name for your bot. It must end in `bot`. Like this, for example: TetrisBot or tetris_bot.
You: SMTFirstBot
BotFather: Done! Congratulations on your new bot. You will find it at telegram.me/SMTFirstBot. You can now add a description, about section and profile picture for your bot, see /help for a list of commands. By the way, when you've finished creating your cool bot, ping our Bot Support if you want a better username for it. Just make sure the bot is fully operational before you do this.Use this token to access the HTTP API:
2764xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
Once you’ve created a bot and received your authorization token, head down to the Bot API manual to see what you can teach your bot to do. The Bot API is an HTTP-based interface created for developers keen on building bots for Telegram.
Changing the SMTFirstBot’s description
You: /setdescription
BotFather: Choose a bot to change description.
You: @SMTFirstBot
BotFather:OK. Send me the new description for the bot. People will see this description when they open a chat with your bot, in a block titled ‘What can this bot do?’.
You: This is a simple and my first BOT.
BotFather: Success! Description updated.
Changing the About section of the SMTFirstBot
You: /setabouttext
BotFather: Choose a bot to change the about section.
You: @SMTFirstBot
BotFather: OK. Send me the new ‘About’ text. People will see this text on the bot’s profile page and it will be sent together with a link to your bot when they share it with someone.
You: My first Telegram Bot.
BotFather: Success! About section updated.
Changing the SMTFirstBot’s Profile Photo
You: /setuserpic
BotFather: Choose a bot to change profile photo.
You: @SMTFirstBot
BotFather: OK. Send me the new profile photo for the bot.
You: [ Photo ]
BotFather: Success! Profile photo updated.
Changing the privacy of SMTFirstBot
You: /setprivacy
BotFather: Choose a bot to change group messages settings.
You: @SMTFirstBot
BotFather: 'Enable' - your bot will only receive messages that either start with the '/' symbol or mention the bot by username.
'Disable' - your bot will receive all messages that people send to groups.
Current status is: ENABLED
You: Disable
BotFather: Success! The new status is: DISABLED.
Authorizing your bot
Each bot is given a unique authentication token when it was created. The token looks something like 123456:ABC-DEF1234ghIkl-zyx57W2v1u123ew11, but they simply use <token> in their manual.
Talking to your bot
Once you create a bot and have obtained the token you can start to interact with your bot.
Making requests
All queries to the Telegram Bot API must be served over HTTPS and need to be presented in this form: https://api.telegram.org/bot<token>/METHOD_NAME. Like this for example:
https://api.telegram.org/bot123456:ABC-DEF1234ghIkl-zyx57W2v1u123ew11/getMe
They support GET and POST HTTP methods. They also support four ways of passing parameters in Bot API requests:
- URL query string
- application/x-www-form-urlencoded
- application/json (except for uploading files)
- multipart/form-data (use to upload files)
The response contains a JSON object, which always has a Boolean field ‘ok’ and may have an optional String field ‘description’ with a human-readable description of the result. If ‘ok’ equals true, the request was successful and the result of the query can be found in the ‘result’ field. In case of an unsuccessful request, ‘ok’ equals false and the error is explained in the ‘description’. An Integer ‘error_code’ field is also returned, but its contents are subject to change in the future.
- All methods in the Bot API are case-insensitive.
- All queries must be made using UTF-8.
You can view a list of method names on the official api documentation.
getMe method
Let’s say we want to retrieve basic information about the newly created bot, we need to use the getMe method.
https://api.telegram.org/bot123456:ABC-DEF1234ghIkl-zyx57W2v1u123ew11/getMe
The response I got was:
{"ok":true,"result":{"id":276401636,"first_name":"SMTFirst","username":"SMTFirstBot"}}
getUpdates method
There are two mutually exclusive ways of receiving updates for your bot — the getUpdates method (which we shall use below) and Webhooks (we shall use in a different article) on the other. Incoming updates are stored on the server until the bot receives them either way, but they will not be kept longer than 24 hours.
If we need the chat_id of a person sending a message to our bot, then use the getUpdates method.
https://api.telegram.org/bot123456:ABC-DEF1234ghIkl-zyx57W2v1u123ew11/getUpdates
The response I got was:
{"ok":true,"result":[{"update_id":839499903,
"message":{"message_id":12,"from":{"id":246367260,"first_name":"Satish","last_name":"Talim","username":"IndianGuru"},"chat":{"id":246367260,"first_name":"Satish","last_name":"Talim","username":"IndianGuru","type":"private"},"date":1473914771,"text":"Hello SMTFirstBot"}}]}
As you can see my chat_id is 246367260
sendMessage method
Use method sendMessage to send text messages from the Bot to the user using his/her chat_id.
https://api.telegram.org/bot123456:ABC-DEF1234ghIkl-zyx57W2v1u123ew11/sendMessage?chat_id=246367260&text="Hello from SMTFirstBot"
The response I got was:
{"ok":true,"result":{"message_id":13,"from":{"id":276401636,"first_name":"SMTFirst","username":"SMTFirstBot"},"chat":{"id":246367260,"first_name":"Satish","last_name":"Talim","username":"IndianGuru","type":"private"},"date":1473915564,"text":"\"Hello from SMTFirstBot\""}}
Here’s the Go code to get a chat_id and then send a message to this chat_id from MyFirstBot.
Program myfirstbot.go
That’s it. Have fun.
Ten articles before and after
Full Tutorial On How to Create and Deploy a Telegram Bot using Python – Best Telegram
QuestBETS is launching the first Telegram based sports betting in few days! – Best Telegram
JavaScript FullStack – Como criar um Chatbot no Telegram em NodeJS – Best Telegram
Create an automated bot on Telegram without coding, using Zapier and Paquebot. – Best Telegram
Operational Telegram. Telegram, the encrypted messaging app… – Best Telegram
A recap of last week live Telegram session with COTI’s CEO Shahaf Bar-Gefen – Best Telegram
Pundi X integrates Telegram chat and adds Crypto Gift feature for its XWallet app – Best Telegram
Telegram VS Whatsapp. Which one should you choose? – Best Telegram
My Pain Points writing a Telegram Bot (in Python) – Best Telegram